





Installation
VS Code & PlatformIO:
- Install VS Code
- Install PlatformIO
- Create a new Project
- Use the Library Manager to add the library to your project or add
sinricpro/SinricPro
manually to your lib_deps
in platformio.ini
.
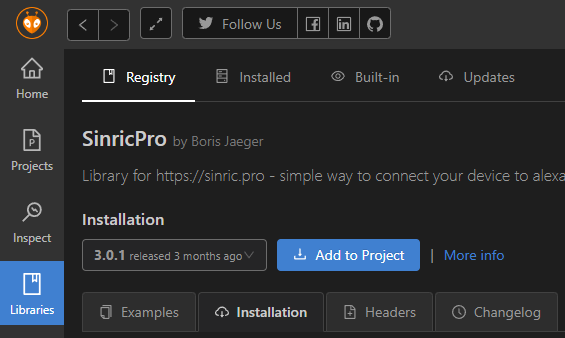
ArduinoIDE
- Open Library Manager (Tools / Manage Libraries)
- Search for SinricPro and click Install
- Repeat step 2 for all dependent libraries!
- Open example in ArduinoIDE (File / Examples / SinricPro / ...)
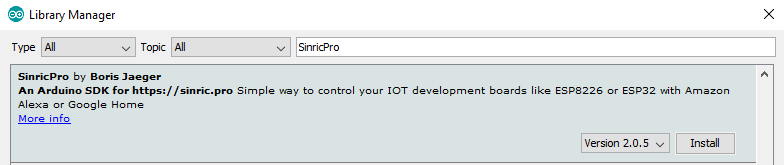
Dependencies
- ArduinoJson by Benoit Blanchon (minimum Version 7.0.3)
- WebSockets by Markus Sattler (minimum Version 2.4.0)
Full user documentation
Please see here for full user documentation
Examples
See examples on GitHub
Usage
Include SinricPro-Library (SinricPro.h) and SinricPro-Device-Libraries (eg. SinricProSwitch.h)
{C++}
#include <SinricPro.h>
#include <SinricProSwitch.h>
Define your credentials from SinricPro-Portal (portal.sinric.pro)
{C++}
#define APP_KEY "YOUR-APP-KEY" // Should look like "de0bxxxx-1x3x-4x3x-ax2x-5dabxxxxxxxx"
#define APP_SECRET "YOUR-APP-SECRET" // Should look like "5f36xxxx-x3x7-4x3x-xexe-e86724a9xxxx-4c4axxxx-3x3x-x5xe-x9x3-333d65xxxxxx"
#define SWITCH_ID "YOUR-DEVICE-ID" // Should look like "5dc1564130xxxxxxxxxxxxxx"
Define callback routine(s)
{C++}
bool onPowerState(const String &deviceId, bool &state) {
Serial.printf("device %s turned %s\r\n", deviceId.c_str(), state?"on":"off");
return true; // indicate that callback handled correctly
}
In setup()
{C++}
// create and add a switch to SinricPro
SinricProSwitch& mySwitch = SinricPro[SWITCH_ID];
// set callback function
mySwitch.onPowerState(onPowerState);
// startup SinricPro
SinricPro.begin(APP_KEY, APP_SECRET);
In loop()
{C++}
SinricPro.handle();
How to add a device?
Syntax is
{C++}
DeviceType& myDevice = SinricPro[DEVICE_ID];
Example
{C++}
SinricProSwitch& mySwitch = SinricPro["YOUR-SWITCH-ID-HERE"];
How to retrieve a device for sending an event?
Syntax is
{C++}
DeviceType& myDevice = SinricPro[DEVICE_ID];
Example
{C++}
SinricProDoorbell& myDoorbell = SinricPro["YOUR-DOORBELL-ID-HERE"];
myDoorbell.sendDoorbellEvent();
How to send a push notification?
{C++}
SinricProSwitch& mySwitch = SinricPro[SWITCH_ID];
mySwitch.sendPushNotification("Hello SinricPro!");
Device Types
Other
* <a href="https://github.com/sinricpro/esp8266-esp32-sdk/tree/master/examples/Health">Health</a>
Licensing and Credits
- The Arduino IDE is developed and maintained by the Arduino team. The IDE is licensed under GPL.
- ArduinoJson is licensed under the MIT.
- WebSockets is licensed under the GNU LGPL.
- The PlatformIO is developed and maintained by the PlatformIO team. The Core is licensed under Apache License 2.0.
Support for other boards
https://github.com/sinricpro/arduino-variants-sdk
Join the community!
Join us on our Official Discord Server!