
"" "" "" ""



Note
- Use the latest ESP8226/ESP32/RP2040 Arduino Core!
- Use the latest WebSocktes library!
- Use the latest ArduinoJson library!
Installation
VS Code & PlatformIO:
- Install VS Code
- Install PlatformIO
- Install SinricPro library by using Library Manager
- Use included platformio.ini file from examples to ensure that all dependent libraries will installed automaticly.
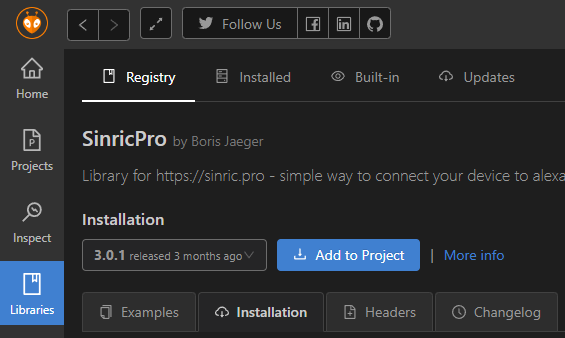
ArduinoIDE
- Open Library Manager (Tools / Manage Libraries)
- Search for SinricPro and click Install
- Repeat step 2 for all dependent libraries!
- Open example in ArduinoIDE (File / Examples / SinricPro / ...)
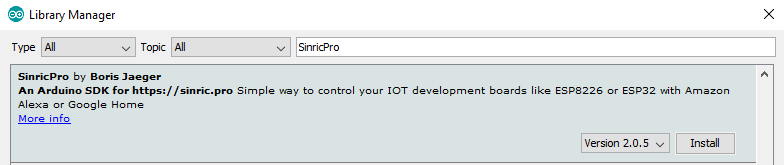
Dependencies
ArduinoJson by Benoit Blanchon (minimum Version 6.12.0)
WebSockets by Markus Sattler (minimum Version 2.4.0)
Full user documentation
Please see here for full user documentation
Examples
See examples on GitHub
Usage
Include SinricPro-Library (SinricPro.h) and SinricPro-Device-Libraries (eg. SinricProSwitch.h)
++
#include <SinricPro.h>
#include <SinricProSwitch.h>
Define your credentials from SinricPro-Portal (portal.sinric.pro)
++
#define APP_KEY "YOUR-APP-KEY"
#define APP_SECRET "YOUR-APP-SECRET"
#define SWITCH_ID "YOUR-DEVICE-ID"
Define callback routine(s)
++
bool onPowerState(const String &deviceId, bool &state) {
Serial.printf("device %s turned %s\r\n", deviceId.c_str(), state?"on":"off");
return true;
}
In setup()
++
SinricProSwitch& mySwitch = SinricPro[SWITCH_ID];
mySwitch.onPowerState(onPowerState);
SinricPro.
begin(APP_KEY, APP_SECRET);
void begin(String appKey, String appSecret, String serverURL="ws.sinric.pro")
Initializing SinricProClass to be able to connect to SinricPro Server.
Definition SinricPro.h:176
In loop()
++
void handle()
Handles communication between device and SinricPro Server.
Definition SinricPro.h:234
How to add a device?
Syntax is
++
DeviceType& myDevice = SinricPro[DEVICE_ID];
Example
++
SinricProSwitch& mySwitch = SinricPro["YOUR-SWITCH-ID-HERE"];
Example 2 (alternatively)
++
SinricProSwitch& mySwitch = SinricPro.add<SinricProSwitch>("YOUR-SWITCH-ID-HERE");
How to retrieve a device for sending an event?
Syntax is
++
DeviceType& myDevice = SinricPro[DEVICE_ID];
Example 1
++
SinricProDoorbell& myDoorbell = SinricPro["YOUR-DOORBELL-ID-HERE"];
myDoorbell.sendDoorbellEvent();
Example 2 (alternatively)
++
SinricPro["YOUR-DOORBELL-ID-HERE"].as<SinricProDoorbell>().sendDoorbellEvent();
How to send a push notification?
++
SinricProSwitch& mySwitch = SinricPro[SWITCH_ID];
mySwitch.sendPushNotification("Hello SinricPro!");
Devices
- Switch
- Dimmable Switch
- Light
- TV
- Speaker
- Thermostat
- Fan (US and non US version)
- Lock
- Doorbell
- Temperaturesensor
- Motionsensor
- Contactsensor
- Windows Air Conditioner
- Interior Blinds
- Garage Door
* Custom devices